例外でて進まなかったのでですが、いけたので続き記載します.
例外対策
Permission.HasUserAuthorizedPermission(Permission.Camera)
↑を使用しているところで例外が発生していたので、この部分を削除した場合にどうなるかチェックしたところ・・・・
(コード修正部分)
/// <summary>
/// 使用できるかどうかチェックチェック
/// </summary>
/// <returns></returns>
private bool CheckCamera()
{
try
{
//// カメラの使用が許可チェック
//if (!Permission.HasUserAuthorizedPermission(Permission.Camera))
//{
// // カメラの使用許可リクエスト
// Permission.RequestUserPermission(Permission.Camera, m_CallBack);
// // カメラ使用チェック
// m_CameraCheck = true;
// // ボンタン使えなくする
// m_Button.gameObject.SetActive(false);
// // まだカメラ使用OKされていない状態へ
// return false;
//}
//else
//{
// // カメラ使用OK
// return true;
//}
return true;
}
catch(Exception e)
{
m_ResultText.text = e.Message;
return false;
}
}
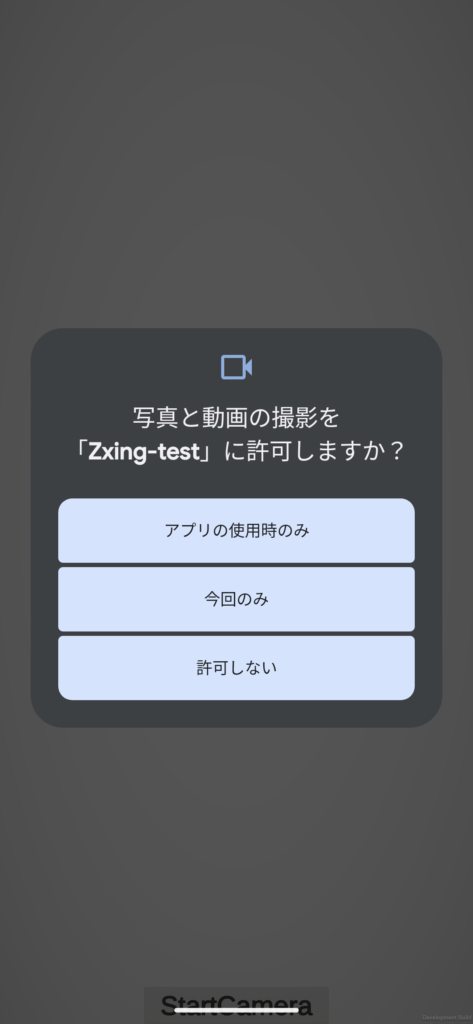
え・・・
ちゃんと使用許可画面出るやんけ・・・・
ただ現状だと許可しないを押されたときのコールバックの設定ができていないので
それは後々に調べるとして、これで実際にカメラからQRコードを読めるか確認します。
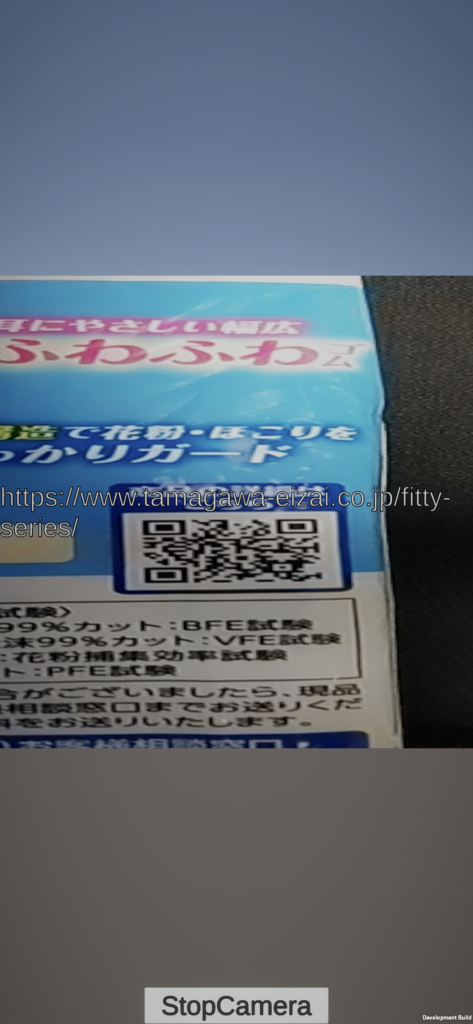
このような形でQRコードのURLが取得できます。
1次元バーコードも読んでみる
ZXingはQRコードだけではなく1次元のバーコードも読み込めるはずなので、その対応をします。
まずはQRコードリーダーを変更します。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using ZXing;
/// <summary>
/// QRコード読み込み
/// </summary>
public class QRCodeReder : SingletonMonoBehaviour<QRCodeReder>
{
/// <summary>
/// 読み込む種類
/// </summary>
private readonly List<BarcodeFormat> BarcodeFormats = new List<BarcodeFormat>
{
BarcodeFormat.CODABAR,
BarcodeFormat.CODE_39, // 1Dバーコード 数字、アルファベットといくつかの記号の合計43個
BarcodeFormat.CODE_93,
BarcodeFormat.CODE_128, // 1Dバーコード アスキーコード128文字
BarcodeFormat.EAN_8, // 1Dバーコード 基本形 8桁
BarcodeFormat.EAN_13, // 1Dバーコード 基本形 13桁
BarcodeFormat.UPC_A, // 1Dバーコード 北米 12桁
BarcodeFormat.UPC_E, // 1Dバーコード 北米 8桁
BarcodeFormat.ITF, // 段ボールに印刷されている物流商品コード用
BarcodeFormat.DATA_MATRIX, // 2D用
BarcodeFormat.QR_CODE, // 2D用
};
/// <summary>
/// 読み込み用
/// </summary>
private BarcodeReader m_Reader;
// Start is called before the first frame update
void Start()
{
m_Reader = new BarcodeReader()
{
AutoRotate = true, // 回転ON
Options = new ZXing.Common.DecodingOptions()
{
TryHarder = true, // より深く見る?(精度上げている?)
TryInverted = true, // 自動回転ON
PossibleFormats = BarcodeFormats, // 読み取るフォーマット
}
};
}
// Update is called once per frame
void Update()
{
}
/// <summary>
/// TextureからのQRコード読み込み
/// </summary>
/// <param name="tex">テクスチャ</param>
/// <returns>読み込んだテクスチャの文字列</returns>
public string ReadQRCodeTexture(Texture2D tex,bool AutoRotate = true , bool TryInverted = true)
{
m_Reader.AutoRotate = AutoRotate;
m_Reader.Options.TryInverted = TryInverted;
// 読み込み
ZXing.Result r = m_Reader.Decode(tex.GetPixels32(), tex.width, tex.height);
return r != null ? r.Text : string.Empty;
}
/// <summary>
/// WebCamTextureからのQRコード読み込み
/// </summary>
/// <param name="tex">テクスチャ</param>
/// <returns>読み込んだテクスチャの文字列</returns>
public string ReadQRCodeWebCamTexture(WebCamTexture tex, bool AutoRotate = true, bool TryInverted = true)
{
m_Reader.AutoRotate = AutoRotate;
m_Reader.Options.TryInverted = TryInverted;
// 読み込み
ZXing.Result r = m_Reader.Decode(tex.GetPixels32(), tex.width, tex.height);
return r != null ? r.Text : string.Empty;
}
}
BarcodeFormats を定義してデコードするフォーマットを設定します。
BarcodeReaderもクラス変数として定義してStart時に初期化して使いまわしています。
各種読み込み用の関数に回転をどうするかの変数を定義しているのは、
回転部分を有効にすると重くなるのでoffにできるようにしています。
(この時点でも毎フレームデコードしているので重いですが・・・・)
この状態で通常のバーコードを読むと・・・
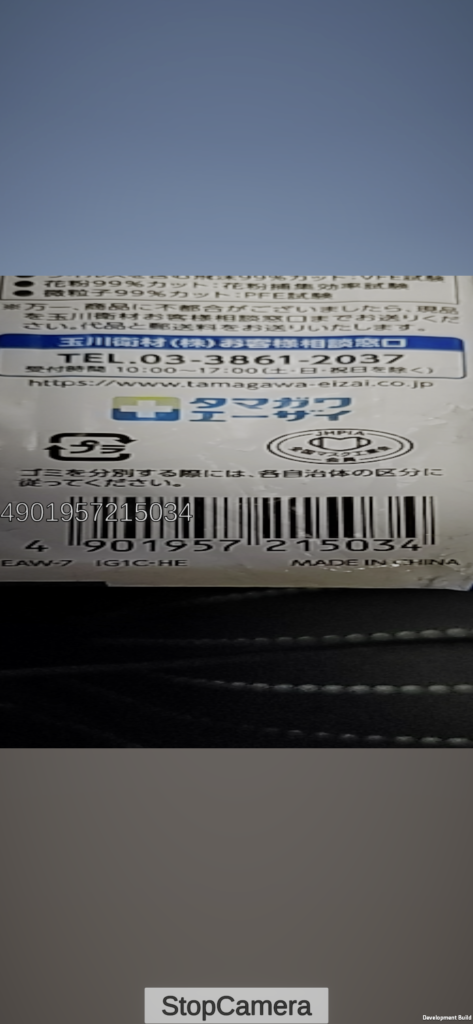
このような感じでバーコードの数値が読めます。
あとは
// Update is called once per frame
void Update()
{
if(m_StartCamera)
{
// TODO ここマイフームやっているから重い どうにかした方が良い
m_ResultText.text = m_QRCodeReder.ReadQRCodeWebCamTexture(m_webCamTexture,false,false);
}
}
この部分の毎フレームチェックしているところをどうにかできればまともに使えそうですね・・・
コメント